Angular is a leading framework for building dynamic web applications, developed and maintained by Google. To truly master Angular, developers need to understand its core concepts and features. In this article, we’ll cover the top 10 Angular concepts you must know to become proficient in Angular development.
1. Angular Components
Components are the fundamental building elements of any Angular application. They define the HTML templates, styles, and logic that render the user interface.
Key points
– Component Decorator: Use the @Component decorator to define metadata for your components.
– Component Lifecycle hook: Learn lifecycle hooks like ngOnInit and ngOnDestroy to manage component behaviour.
2. Angular Modules
Modules help organize your Angular application into cohesive blocks of functionality. Every Angular app starts with at least one module, the root module, but large applications benefit from feature modules.
Key points
– NgModule Decorator: Use the @NgModule decorator to declare components, directives, pipes, and services.
– Lazy loading: Improve performance by loading feature modules only when needed.
3. Data binding in Angular
Data binding synchronizes data between the model and view. Angular supports several types of data binding, each serving different purposes.
Key points
– Interpolation: Bind data from the component class to the template using {{ }}.
– Property binding: Use [ ] to bind element properties to component properties.
– Event binding: Use ( ) to bind events to component methods.
– Two way binding: Use [(ngModel)] for two-way data binding.
Also read: What are Javascript callbacks
4. Directives in Angular
Directives are classes that add behaviour to elements in Angular applications. They help manipulate the DOM and extend HTML.
Key points
– Attribute Directives: Change the appearance or behaviour of elements (e.g., ngClass, ngStyle ).
– Structural Directives: Manipulate the DOM by adding or removing elements (e.g., *ngIf, *ngFor ).
5. Services and Dependency Injection
Services in Angular are used to share data and functionality across components. Angular’s dependency injection system allows you to inject services into components and other services.
Key points
– Creating services: Use the @Injectable decorator to define a service class.
– Providing services: Register services with Angular’s injector, either at the module or component level.
6. Angular Routing
Routing enables navigation between different views or components. The Angular Router module provides a robust system for defining and managing routes.
Key points
– Router module: Import RouterModule and configure routes.
– Router parameters: Pass parameters to routes to create dynamic views.
– Route guards: Implement route guards ( CanActivate, CanDeactivate ) to control access to routes.
7. Angular Forms
Angular provides two approaches to building forms: Template-driven and Reactive forms. Each approach suits different needs and complexities.
Key points
– Template-driven forms: Use directives and template syntax for simple forms.
– Reactive forms: Use a model-driven approach for more complex forms.
8. Angular Pipes
Pipes transform data in templates. They are simple functions that take in data, process it, and return the transformed data.
Key points
– Built-in Pipes: Utilize Angular’s built-in pipes like DatePipe, UpperCasePipe, and CurrencyPipe.
– Custom Pipes: Create custom pipes for specific data transformations.
9. HttpClient Module
The `HttpClient` module allows communication with backend services over HTTP. It provides an easy-to-use API for making HTTP requests and handling responses.
Key points
– Making Requests: Use methods like GET, POST, PUT, and DELETE to interact with APIs.
– Interceptors: Implement HTTP interceptors to modify requests or responses, such as adding authentication tokens.
10. State Management in Angular
Managing the state in large Angular applications can be challenging. Tools like NgRx provide a structured way to manage application state using a Redux-like architecture.
Key points
– Store: Centralize application state in a single store.
– Actions and Reducers: Use actions to describe state changes and reducers to handle these changes.
– Effects: Manage side effects, such as asynchronous operations, in a clean and maintainable way.
Conclusion
Mastering these top 10 Angular concepts is essential for becoming a proficient Angular developer. By thoroughly understanding components, modules, data binding, directives, services, routing, forms, pipes, HTTP client, and state management, you’ll be well-equipped to build robust, scalable, and maintainable Angular applications. Dive into each concept, practice regularly, and you’ll soon master Angular development.
By focusing on these key concepts, you can significantly enhance your Angular skills and become an expert in Angular development. Happy coding!
References
About Author
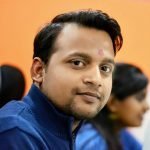
Prabhat Giri
Software engineer (@Prabhat Giri)Hi thanks for reading my blog. I am a software professional who is also passionate about business and travelling.
Popular topicsCareer Javascript Web Development Job Preparation Angular Geekncoder